Automate Tasks Using Shell Script and Cron Job
Good programmers doesn't just find the best solutions to a problem, they also find the easiest way to solve it.
Intro
Good programmers doesn't just find the best solutions to a problem, they also find the easiest way to solve it.
Automating tasks is one of the best way to make your life and work easy. Some programmers I know automates even the smallest tasks on their daily routines. E.g. sending an email every morning to greet someone from work.
We can achieve automation by using shell scripting and executing it on a given time using a cron job.
For our example, we will create a very basic task. We will print the words "Random Code Tips!" every 10 minutes and save it to a log file.
Shell Script
Open your terminal and create a file called my-script.sh
in a directory of your choice.
Copy the code below:
#!/bin/sh
# This is a comment!
echo "Random Code Tips!"
- The first line
#!/bin/sh
means that the script should be run using bash. More information about this. - The second line is a comment. Comments starts with
#
in shell scripting. - The third line, the echo command will print the words "Random Code Tips!".
Before testing our newly created script, we must add an execute permission to it. Open a terminal to the directory where your script is and enter the command chmod +x ./my-script.sh
This command means you are giving yourself (the user of the machine) permission to run the script. To learn more about Unix file permissions you can read this.
Now go ahead and test your script. On the same terminal type the command ./my-script.sh
and press ENTER.
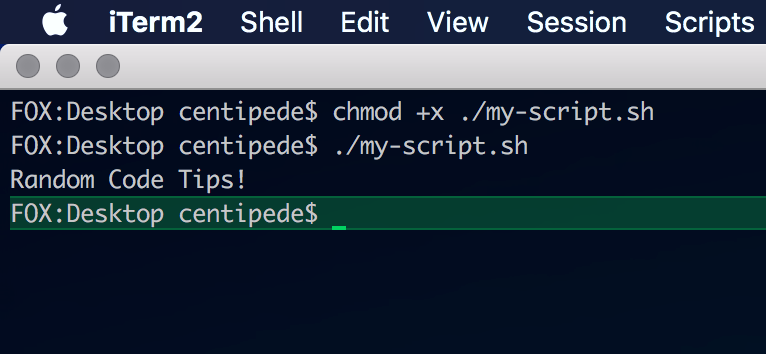
It works! The words "Random Code Tips!" has been printed successfully.
Now, how do we run without manually opening the terminal and typing the command ./my-script.sh
? That's where cron can help us.
The software utility cron is a time-based job scheduler in Unix-like computer operating systems.https://en.wikipedia.org/wiki/Cron
So basically... an alarm clock. But cooler.
To start a cron job, open a terminal and type the command crontab -e
. crontab
or Cron Table is a file containing all the jobs that needs to be executed. The flat -e
means edit. So we are just saying "I want to edit the cron table".
This command will open a text editor in my case vim
. Now type the following code and save it.
*/10 * * * * /path/to/my-script.sh >> /path/to/my-script.log
I will break down this and explain every part. *
- The first part
*/10 * * * *
represents the time on which the will be executed. So the*/10
represents the minutes "minutes". This one means "every 10 minutes". See below: - The second is the absolute path
/path/to/my-script.sh
is just the path to ourmy-script.sh
file. - The
>>
means we will write (append) every activities that happens to ourmy-script.sh
to the last part: /path/to/my-script.log
the absolute path to themy-script.log
file. We will see everything that has been logged in by ourmy-script.sh
here.
# From https://en.wikipedia.org/wiki/Cron
#
# ┌───────────── minute (0 - 59)
# │ ┌───────────── hour (0 - 23)
# │ │ ┌───────────── day of the month (1 - 31)
# │ │ │ ┌───────────── month (1 - 12)
# │ │ │ │ ┌───────────── day of the week (0 - 6) (Sunday to Saturday;
# │ │ │ │ │ 7 is also Sunday on some systems)
# │ │ │ │ │
# │ │ │ │ │
# * * * * * command to execute
Our shell script be running now every 10 minutes.
You can use this technique create more complex tasks that will make your work easier. Here are some examples:
- Server health checker
- Scheduled email
Helpful Resources
- crontab.guru
- https://en.wikipedia.org/wiki/Cron
- https://www.shellscript.sh/
Now you know how to create automated tasks. Work smart!
Happy Coding!