Unpacking the package.json - Explaining the most commonly used parts of NPM's package.json
package.json file is an important part of any Node.js project, as it contains information about the project, its dependencies, and other metadata. In this blog post, we'll go through all the different parts of a package.json file and explain what they do.
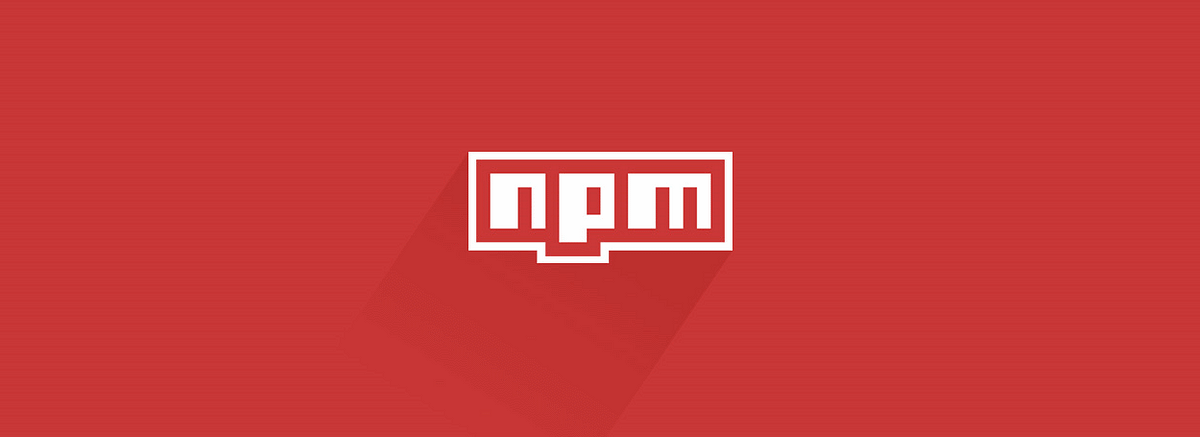
Hello! If you've ever worked with Node.js before, you've probably come across a file called package.json
. This file is an important part of any Node.js project, as it contains information about the project, its dependencies, and other metadata. In this blog post, we'll go through all the different parts of a package.json
file and explain what they do.
First, let's take a look at what a package.json
file looks like:
{
"name": "my-node-project",
"version": "1.0.0",
"description": "A sample Node.js project",
"main": "index.js",
"dependencies": {
"express": "^4.17.1",
"dotenv": "^10.0.0"
},
"devDependencies": {
"nodemon": "^2.0.14"
},
"scripts": {
"start": "node index.js",
"dev": "nodemon index.js"
},
"repository": {
"type": "git",
"url": "https://github.com/username/my-node-project.git"
},
"author": "Your Name",
"license": "MIT"
}
As you can see, a package.json
file is a JSON object that contains a number of different keys. Let's go through each of them one by one.
"name"
The "name"
key is used to specify the name of the project. This name must be unique on the npm registry, so it's a good idea to use a name that is specific to your project. For example, if your project is a chat application, you might name it "my-chat-app".
"name": "my-chat-app"
"version"
The "version"
key is used to specify the version of your project. It's a good idea to use semantic versioning (semver) for your project, which consists of three numbers separated by dots: major.minor.patch
. For example, if your project is at version 1.2.3 and you make a backwards-incompatible change, you would increment the major version to 2.0.0.
"version": "1.2.3"
"description"
The "description"
key is used to provide a brief description of your project. This can be helpful for people who are browsing the npm registry and are trying to decide whether to use your project or not.
"description": "A sample Node.js project"
"main"
The "main"
key is used to specify the main entry point of your project. This is the file that will be executed when someone runs your project. By convention, this file is usually named index.js
.
"main": "index.js"
"dependencies"
The "dependencies"
key is used to specify the dependencies that your project has. These are other Node.js modules that your project depends on in order to run. Dependencies are installed using npm install
, which reads the "dependencies"
key and downloads the specified modules from the npm registry.
"dependencies": {
"express": "^4.17.1",
"dotenv": "^10.0.0"
}
In this example, our project depends on the express
and dotenv
modules, which will be downloaded and installed when we run npm install
.
"devDependencies"
The "devDependencies"
key is similar to "dependencies"
, but it's used to specify modules that are only needed during development. These modules are not required for the production version of your project, so you can save some space and speed up installation times by separating them out into this key. They are installed using npm install
with the --dev
flag.
"devDependencies": {
"nodemon": "^2.0.14"
}
In this example, our project has a development dependency on nodemon
, which is a utility that automatically restarts the server when changes are made.
"scripts"
The "scripts"
key is used to define commands that can be run using npm run
. These commands can be used to start the server, run tests, build the project, and more.
"scripts": {
"start": "node index.js",
"dev": "nodemon index.js"
}
In this example, we have defined two scripts: "start"
and "dev"
. "start"
will start the server using node index.js
, while "dev"
will start the server using nodemon index.js
.
"repository"
The "repository"
key is used to specify the location of the project's source code repository. This can be helpful for people who want to contribute to your project or report issues.
"repository": {
"type": "git",
"url": "https://github.com/username/my-node-project.git"
}
In this example, our project is hosted on GitHub and the repository URL is "https://github.com/username/my-node-project.git"
.
"author"
The "author"
key is used to specify the author of the project.
"author": "Your Name"
"license"
The "license"
key is used to specify the license that the project is released under. There are many different open source licenses to choose from, so be sure to choose one that fits your needs.
"license": "MIT"
In this example, our project is released under the MIT license.
That's it! Those are all the different parts of a package.json
file. By including this file in your Node.js project, you can provide important information about your project and its dependencies, as well as define scripts that make it easier to work with.
About the author
Joff Tiquez, hailing from Manila, Philippines, is the individual behind the establishment of OSSPH. He is a web developer who strongly supports open source and has been overseeing projects like Vue Stripe for an extended period. To get in touch with Joff, you can visit https://bento.me/jofftiquez.