13 Advanced Git Commands You Need to Know
Git is incredibly powerful and flexible, but it can also be complex and overwhelming, especially for new users. While the basic Git commands are enough to get you started, there are many lesser-known commands that can help you take your Git skills to the next level.
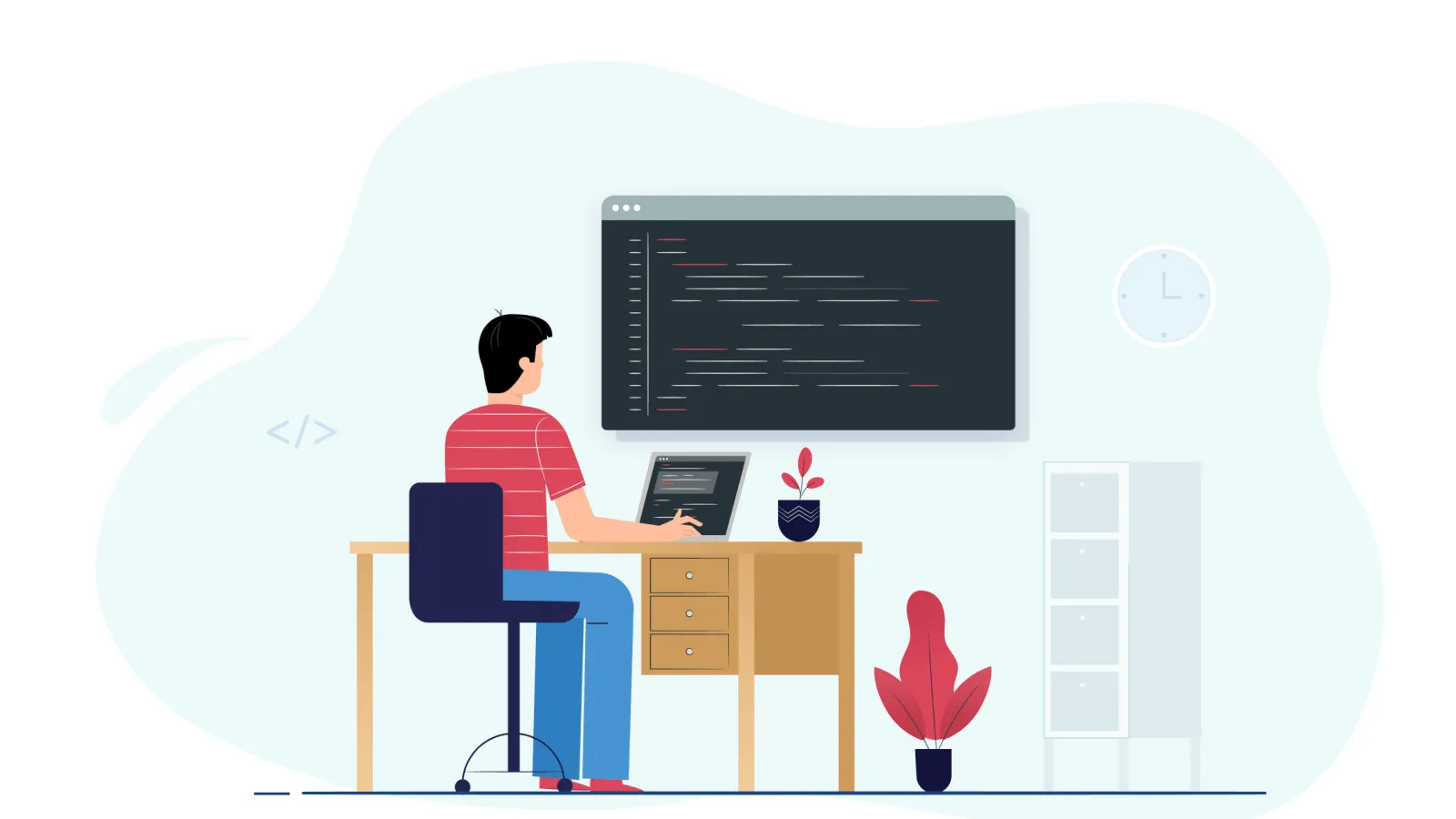
Git is an essential tool for version control in software development. While most developers are familiar with basic Git commands like commit, push, and pull, there are many lesser-known commands that can make working with Git much easier and more efficient. In this article, we'll explore 13 useful Git commands that you might not know about but can greatly improve your workflow. From managing your repository history to finding and fixing bugs, these commands can help you in enhancing your Git skills and streamline your development process.
Git Archive
Used for: Creating a compressed archive of Git repository
Git archive allows you to create a compressed archive of the files in your Git repository at a specific commit. You can use it to export a specific version of your code without the Git history. To use the command, simply type git archive --format=<format> <commit> -o <filename>
in your terminal, where <format>
is the desired file format, <commit>
is the commit you want to archive, and <filename>
is the desired output file name. This command is useful when you want to share your code with someone who doesn't use Git or when you want to create a ZIP or TAR archive of your code without the Git history.
Git Bisect
Used for: Finding the commit that introduced a bug
Git Bisect is a command that can help you pinpoint the exact commit that introduced the bug, saving you time and effort in the debugging process. Git Bisect is a command in Git that can help you pinpoint the commit that introduced the problem. To use Git Bisect, you first need to know a good commit (one where the issue doesn't exist) and a bad commit (one where the issue does exist). Then, you can run git bisect start
and git bisect bad
to mark the current commit as bad. Then, you can check out an older commit and mark it as good with git bisect good
. Git Bisect will then automatically narrow down the range of commits to check, asking you to test each one until you find the commit that introduced the issue. Git Bisect can be a powerful command for debugging complex problems in your code and can save you a lot of time and effort in the long run.
Git Blame
Used for: Showing who last modified each line of a file
Git Blame is a command that allows you to see who made each change to a file, making it easy to track down the original author of a particular line of code. To use Git Blame, simply run the command git blame <file>
in your terminal. This will display each line of the file, along with the commit hash, author, and date of the last change made to that line. Git Blame can be especially useful when working with large codebases or collaborating with other developers, allowing you to quickly identify who made each change and why. By using Git Blame, you can gain a better understanding of the code you're working with and make more informed decisions about how to modify it.
Git Cherry Pick
Used for: Applying a specific commit from one branch to another
Git cherry-pick is a useful command when applying changes from one branch to another without merging the entire branch. It can be helpful in situations where you need to apply a hotfix to a production branch or when you want to move specific changes between branches. Simply use the command git cherry-pick <commit-hash>
followed by the hash of the commit you want to pick, and it will apply the changes to your current branch. Just be careful when cherry-picking commits, as it can lead to conflicts if there are conflicting changes between the two branches. So always make sure to review and test your changes thoroughly before pushing them to production.
Git Clean
Used for: Removing untracked files from the working tree
If you have files in your working directory that you don't want or need, Git Clean can help you out. Git Clean is a command that allows you to remove untracked files from your working directory. These could be temporary files, build artifacts, or other files that you don't need. Using Git Clean is easy - just navigate to the directory where you want to clean up and run the command git clean
. You can also use options like -n
to preview which files will be deleted before actually deleting them, and -d
to delete untracked directories as well as files. Git Clean is useful for keeping your working directory clean and organized. Just be careful not to accidentally delete any important files!
Git Diff
Used for: Showing differences between commits, branches, and files
Git diff allows you to compare the changes between two commits or two branches. It shows the differences between the files, highlighting the lines that were added or removed. Simply type git diff <commit1><commit2>
in your terminal, where <commit1>
and <commit2>
are the two commits you want to compare. This command is useful when you want to review your changes before committing them, or when you want to see the changes made by someone else on your team. It's also helpful when you want to troubleshoot issues by comparing different versions of your code.
Git Grep
Used for: Searching for text in files and commits
Git grep is a command that lets you search for specific text or patterns within your repository. This can be helpful when you need to find all instances of a particular function, variable, or string across your project. It's also useful for debugging or fixing bugs. To use git grep, simply run the command followed by the search term, like git grep <search-term>
. The command will then return a list of files that contain the search term, along with the line numbers where it appears. This can be particularly helpful when working on large projects with many files and lines of code.
Git Rebase
Used for: Merging changes from one branch to another
Git rebase is a command that allows you to move your branch to a new base commit. It helps you apply changes made to one branch to another branch, making it easier to keep your branch up-to-date with the latest changes from the main branch. To use the command, simply type git rebase <base>
in your terminal, where <base>
is the commit or branch you want to move your branch to. Git rebase is particularly useful when you want to tidy up your branch's commit history before merging it with the main branch, making your commit history cleaner and easier to follow. However, it's important to use Git rebase with caution, as it can potentially cause conflicts and rewrite the history of your branch.
Git Reflog
Used for: Showing a log of all changes to the repository's references
Git reflog is a command that shows a list of all the actions you've taken in your Git repository, including commits, merges, and resets. It's useful when you've made a mistake and need to undo a previous action or find a lost commit. The reflog contains a detailed history of all the changes you've made to the repository, even if you've deleted or changed branches. With Git reflog, you can easily find the commit you want to restore or undo and recover your lost work. To use git reflog, simply run the command git reflog
in your terminal, and it will display a list of all the changes made to your repository, along with their corresponding commit hash. You can then use the commit hash to restore any lost changes by running the command git checkout <commit-hash>
. Keep in mind that Git reflog only tracks changes made to your local repository and not to any remote repositories, so be sure to push your changes to the remote repository regularly.
Git Revert
Used for: Undoing a previous commit
Git revert is a command that allows you to undo a previous commit without deleting it entirely. It creates a new commit that reverses the changes made in the previous commit, making it easy to fix mistakes or roll back to a previous version of your project. You can use git revert when you want to undo changes that have already been pushed to a shared repository or when you need to fix a bug without affecting other parts of your code. To use git revert, you need to identify the commit you want to undo and run the command git revert <commit-hash>
. This will create a new commit that reverses the changes introduced by the previous commit. It's important to note that git revert doesn't delete any commits or rewrite the commit history, which makes it a safer option compared to other undo methods.
Git Stash
Used for: Temporarily saving changes without committing them
Git stash allows you to save your changes temporarily and switch to another branch or work on something else without committing your changes. Later on, you can apply your saved changes to your current branch or even to a different branch if needed. This is a great way to keep your work organized and avoid losing your progress. To use git stash, simply run the command git stash
to save your changes and git stash apply
to apply them back to your current branch. You can also use git stash pop
to apply your saved changes and remove them from the stash.
Git Submodule
Used for: Including one Git repository within another
If you're working on a project that depends on another project or library, git submodule can be a useful command to keep your code organized. With git submodule, you can include a specific version of another project within your own project, ensuring that both projects' versions are maintained independently. To use git submodule, simply run the command git submodule add [repository URL] [destination folder]
to add a submodule to your project. Then, when you clone your project, you can use git submodule init
and git submodule update
to pull in the external code. This is a great way to manage dependencies and keep your project organized. However, be mindful that git submodules require extra care while managing and can be tricky to use, so make sure to read the documentation carefully before using them.
Git Worktree
Used for: Managing multiple working trees simultaneously
Git worktree is a useful feature that allows you to have multiple working trees or checkouts of the same repository on your local machine. This means you can work on different branches or even different repositories at the same time without having to switch back and forth. You can create a new worktree using the command git worktree add [path] [branch/commit]
and remove it using git worktree remove [path]
. Worktrees can be especially helpful when working on multiple tasks simultaneously, or when collaborating with others on the same project. However, be mindful that each worktree requires disk space and can affect the performance of your machine, so use it only when necessary.
Conclusion
Git has a wide range of commands that can help developers work more efficiently and effectively. By exploring some of the lesser-known commands, developers can unlock new functionalities and improve their overall Git workflow. While the 13 Git commands discussed in this article may not be commonly used, they can prove to be incredibly useful in specific scenarios. We encourage developers to continue exploring and experimenting with Git commands to discover even more ways to streamline their development process.
Please share if you found this article useful. Feel free to respond if you have any thoughts, feedback, or comments.
Until next time, happy coding and learning! Cheers!
About the author
Paula Isabel Signo is a technical writer at OSSPH and a web developer at Point One. In her free time, Paula contributes to various open-source projects, volunteers in the community, and shares her knowledge by writing articles and tutorials. Connect with Paula here to learn more about her work and interests.